Programming assignment required for the application to the program International Game Architecture And Development (IGAD)
Links:
Game only
Game and source code
The game's aim is to get all the red balls in the pockets, while in control of the white ball using the arrow keys. The game is playable but still contains some lazy coding which I plan to fix soon. I will post updates whenever that happens.
C++ and DirectX programming
Thursday, February 13, 2014
Saturday, December 14, 2013
Ball Physics Part 2
In this post the plan is to create multiple balls, program collision detection and collision resolving between the balls.
First thing to do is to declare an array of the Ball structure. To set the number off balls I will use a constant - #define NumOfBalls
Next we initialize the array and set it's size using our constant value.
Next we update the Game class constructor to set values too all members of the array using a loop. I have also given each ball a different size and position.
Next we update all the functions regarding the ball, again looping through every member. The player controlled ball will be ball 0 of the array.
Everything works fine. Now I will get on with the collision detection. The algorithm will be simple - I will create a function which will check if the distance between the centers of each ball is less than the sum of those ball's radii. That function will check just that. Whether the balls collide, it will return true or false respectively.
Next function will be about resolving collision. Since I have not learned my physics lesson about elastic collision, I will just go ahead and switch the two balls' velocities, just to check if my method of detection is working properly. I will use pointers for this function, because I still haven't thought of a more efficient way.
After that at the bottom of the UpdateBall function we create another loop, which checks the other balls if they are colliding with the ball that is currently indexed. This code is highly inefficient but for a small amount of balls onscreen it will do just fine.
Time to compile and see the end result:
As we see the collision system works properly. In the next posts I will try to make it more realistic, with a more complex approach to the collision resolving function.
Source code
Source code
Friday, December 13, 2013
Ball Physics Part 1
Hello and welcome to my blog.
First thing I want to say is that this is the first time I write in a blog, so poor design and confusing imagery could be noticed.
In these series of posts I will attempt to recreate some primitive ball physics using Visual C++.
The software I am using is:
Visual Studio 2012, provided by DreamSpark.
DirectX Sdk, obtained from Microsoft website.
Chili DirectX Framework Source code, consists of code used to create a window and functions used to draw pixels onto the screen.
First we shall begin by creating a structure for our balls. It's purpose is to contain the variables which will be used to determine each ball's characteristics such as current position on the screen, velocity and etc.
I will declare the structure within the "Game" class of the framework, located in the Game.h header file. In it are declared some variables which will define each ball.
To draw each ball we must create a function that takes each ball's coordinates and draws it on the screen accordingly. Since this is strictly a programming blog, only simple geometric shapes will be used. Luckily, the framework mentioned comes with functions for drawing just that. We will use the "DrawCircle" function located in D3DGraphics.cpp file of the framework. It looks like this:
What we notice here are calls of the "PutPixel" function.
It is a function which takes the following values: Position X, Position Y, Red, Green and Blue. Given these values, it draws a pixel on the screen at the given position and colors it with the given values of Red, Green and blue.
Next thing we have to do in order to draw the circle on the screen is to set the initial values of the position of our first ball. We declare an object called ball using our structure. For the first part we will use only one ball.
Next in the "Game" class constructor, located in Game.cpp we set values to our ball's position and size(radius).
Given the fact the created window is 800x600, I set the initial position of our ball at the center of our screen, with radius of 50 pixels.
Next thing to do is to write a function that will give the ball's coordinates to the DrawCircle function and hopefully display it on the screen.
So far it works as it should. Our next step is to add some movement to the ball.
First to the Ball class we add some variables which will help our ball move in a desired fashion.
After that we initialize them with desired values.
Now we have to write some code. First I will create a function which will update the ball's position, given the current position values and the velocity of the ball. I will call it "UpdateBall" and it's purpose will be to update the position of the ball given the current velocity and resolve collision with the edges(borders) of the screen.
I will also create a function which will receive user input and it will effect the ball's velocities accordingly.
After that we call these functions into the main game loop, where they will iterate every frame.
Then compile and run. The result:
What we observe is correct behavior of the ball, except the fact that it bounces on the bottom border of the window indefinitely. In the next part I will attempt to correct this unintended behavior. I will also update the code to support multiple balls onscreen. Apart from that I plan on programming collision detection and elastic collision resolving.
I will soon post links to the source code.
First thing I want to say is that this is the first time I write in a blog, so poor design and confusing imagery could be noticed.
In these series of posts I will attempt to recreate some primitive ball physics using Visual C++.
The software I am using is:
Visual Studio 2012, provided by DreamSpark.
DirectX Sdk, obtained from Microsoft website.
Chili DirectX Framework Source code, consists of code used to create a window and functions used to draw pixels onto the screen.
First we shall begin by creating a structure for our balls. It's purpose is to contain the variables which will be used to determine each ball's characteristics such as current position on the screen, velocity and etc.
I will declare the structure within the "Game" class of the framework, located in the Game.h header file. In it are declared some variables which will define each ball.
To draw each ball we must create a function that takes each ball's coordinates and draws it on the screen accordingly. Since this is strictly a programming blog, only simple geometric shapes will be used. Luckily, the framework mentioned comes with functions for drawing just that. We will use the "DrawCircle" function located in D3DGraphics.cpp file of the framework. It looks like this:
What we notice here are calls of the "PutPixel" function.
It is a function which takes the following values: Position X, Position Y, Red, Green and Blue. Given these values, it draws a pixel on the screen at the given position and colors it with the given values of Red, Green and blue.
Next thing we have to do in order to draw the circle on the screen is to set the initial values of the position of our first ball. We declare an object called ball using our structure. For the first part we will use only one ball.
Next in the "Game" class constructor, located in Game.cpp we set values to our ball's position and size(radius).
Given the fact the created window is 800x600, I set the initial position of our ball at the center of our screen, with radius of 50 pixels.
Next thing to do is to write a function that will give the ball's coordinates to the DrawCircle function and hopefully display it on the screen.
The D3DGraphics class is declared as gfx in the framework.
Next thing we do is call the function inside the "ComposeFrame" function, which iterates every frame and draws whatever is needs to be drawn on the screen.
Then we compile and look at the results:
So far it works as it should. Our next step is to add some movement to the ball.
First to the Ball class we add some variables which will help our ball move in a desired fashion.
After that we initialize them with desired values.
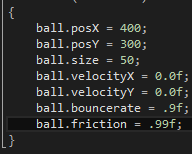
Now we have to write some code. First I will create a function which will update the ball's position, given the current position values and the velocity of the ball. I will call it "UpdateBall" and it's purpose will be to update the position of the ball given the current velocity and resolve collision with the edges(borders) of the screen.
I will also create a function which will receive user input and it will effect the ball's velocities accordingly.
After that we call these functions into the main game loop, where they will iterate every frame.
Then compile and run. The result:
What we observe is correct behavior of the ball, except the fact that it bounces on the bottom border of the window indefinitely. In the next part I will attempt to correct this unintended behavior. I will also update the code to support multiple balls onscreen. Apart from that I plan on programming collision detection and elastic collision resolving.
I will soon post links to the source code.
Subscribe to:
Posts (Atom)